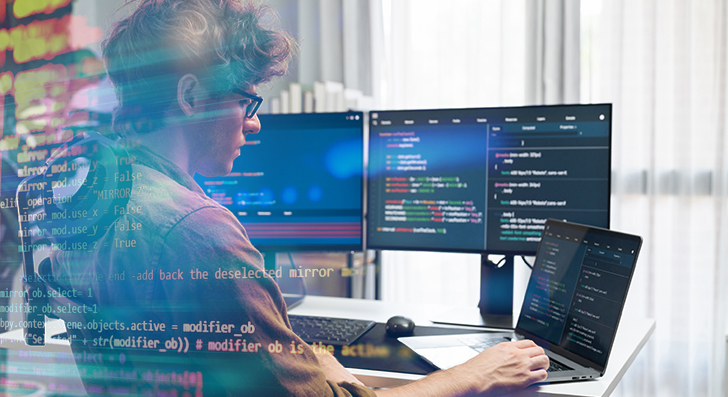
Scalability signifies your software can cope with progress—much more users, additional knowledge, and a lot more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety afterwards. Below’s a clear and realistic guidebook that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it should be part of the plan from the start. Many apps are unsuccessful whenever they grow rapidly because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your procedure will behave under pressure.
Start out by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular design or microservices. These designs split your application into smaller sized, impartial sections. Each module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your databases from working day one. Will it want to manage one million buyers or just a hundred? Choose the proper form—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, even if you don’t need them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t create code that only operates beneath recent ailments. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that guidance scaling, like concept queues or function-driven techniques. These aid your app deal with far more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you are not just planning for achievement—you are reducing potential head aches. A nicely-prepared process is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Database
Deciding on the suitable database is really a key Element of constructing scalable programs. Not all databases are constructed the same, and using the Completely wrong one can gradual you down or maybe result in failures as your application grows.
Start off by knowing your data. Could it be extremely structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely sturdy with relationships, transactions, and regularity. They also assist scaling tactics like study replicas, indexing, and partitioning to manage much more website traffic and information.
In the event your info is a lot more flexible—like consumer exercise logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling huge volumes of unstructured or semi-structured details and may scale horizontally additional very easily.
Also, look at your read through and generate patterns. Do you think you're doing a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a major write load? Explore databases that can manage significant write throughput, or perhaps function-dependent details storage methods like Apache Kafka (for short term knowledge streams).
It’s also good to Feel forward. You might not will need Highly developed scaling attributes now, but selecting a database that supports them implies you gained’t will need to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And generally watch databases performance when you mature.
In a nutshell, the best database is determined by your app’s structure, speed needs, and how you hope it to mature. Choose time to select wisely—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, just about every smaller delay adds up. Poorly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the start.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Resolution if a simple a person will work. Keep your functions small, targeted, and straightforward to check. Use profiling instruments to locate bottlenecks—sites wherever your code requires much too prolonged to run or works by using a lot of memory.
Future, have a look at your database queries. These often sluggish things down a lot more than the code itself. Be sure Every question only asks for the info you really have to have. Stay away from Find *, which fetches every little thing, and as an alternative find particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout significant tables.
Should you detect exactly the same knowledge remaining asked for many times, use caching. Shop the outcome quickly utilizing equipment like Redis or Memcached this means you don’t have to repeat pricey functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one by one, update them in groups. This cuts down on overhead and helps make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done great with 100 records may well crash whenever they have to take care of 1 million.
In short, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when necessary. These methods enable your software keep clean and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and much more visitors. If every little thing goes by means of a single server, it's going to swiftly become a bottleneck. That’s the place load balancing and caching can be found in. Both of these applications enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming traffic throughout various servers. In lieu of a person server executing every click here one of the operate, the load balancer routes consumers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it can be reused immediately. When end users request a similar facts once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to serve it through the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, improves pace, and will make your app extra effective.
Use caching for things which don’t alter generally. And always be sure your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective tools. Collectively, they assist your app manage additional customers, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you'll need equipment that allow your application mature effortlessly. That’s the place cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to rent servers and solutions as you require them. You don’t really have to buy hardware or guess long term capability. When site visitors will increase, it is possible to insert additional methods with just some clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give solutions like managed databases, storage, load balancing, and security tools. You are able to concentrate on building your application in lieu of taking care of infrastructure.
Containers are A different essential Device. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your application works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools suggests you are able to scale rapid, deploy very easily, and recover promptly when issues transpire. If you would like your application to grow with no limits, commence applying these resources early. They help save time, decrease chance, and help you remain centered on building, not fixing.
Watch Everything
When you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place challenges early, and make better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU usage, memory, disk Room, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just monitor your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently problems transpire, and wherever they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on inside your code.
Set up alerts for important problems. For example, if your response time goes higher than a Restrict or maybe a assistance goes down, you must get notified quickly. This will help you resolve concerns quick, frequently before buyers even detect.
Checking is additionally helpful when you make variations. When you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it causes serious hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking will help you maintain your application trustworthy and scalable. It’s not just about spotting failures—it’s about understanding your process and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications need to have a solid foundation. By coming up with very carefully, optimizing sensibly, and using the appropriate applications, you'll be able to Establish apps that increase effortlessly devoid of breaking under pressure. Commence compact, Believe massive, and Establish wise.